This is the easy way to implement webservice.
This example is bottom up approach- from Java - to - WSDL
Step1 : Start Eclipse
Step2 : Open Eclipse-> File -> New Dynamic web project
Step3 : Give project name as you like (ex: test_webservice_server)
Step4 : Select Dynamic web module version as 2.4
Step5 : Select target run time as Apache tomcat version and specify the loaction of
tomcat server installed location.(Ex: c:/tomcat)
Step6 : Click on Finish button.
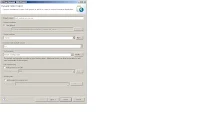
Before creating webservice we need to write the interface and implementation classes to which methods we need to create webservice.
In this example i created one interface called ArthimeticService, having different methods for doing arthemetic operations.
Then created one Class- ArthimeticServiceImpl which implemnts ,ArthimeticService interface.
Now It's time to create webservice.
Step1 : Right Click on the Created project
Step2 : Select New - Other - Webservice
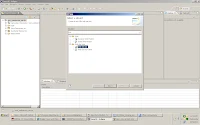
Step3 : Click On Next button.
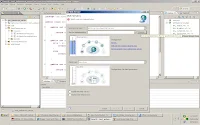
Step4 : Select Service Implementation(Which we already created ex:
ArthimeticServiceImpl)
Step5 : Click on Next- Next -(If screen shows the start server button click on that)- Finish
Once we completed above steps our webservice is ready to use by clients.
We need to check whether our webservice is working or not
Open Created wsdl under Webcontent folder
Go to the <wsdlsoap:address location> copy the value and paste in browser address bar with wsdl like the below.
http://localhost:8888/test_webservice_server/services/ArthimeticServiceImpl?wsdl
Please check the port your server is running(most of the cases it will be 8080)
Once this ready then we can create the client from any system.
Now the time for creating webservice client to access the webservice server.
Step1 : create new - project - dynamic web project (ex: test_webservice_client)
Step2 : Select Dynamic web module version as 2.4.
Step3 : Rigth Click on the created project- New - webservice client.
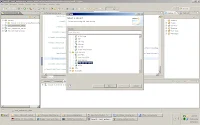
Step4 : Click on Next button.
Step5 : Select the service definition
Step6 : Click on the Browse button
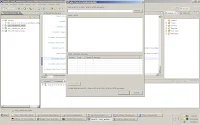
Step7 : Locate the webservice wsdl location
(where wsdl is running : ex http://localhost:8888
/test_webservice_server/services/ArthimeticServiceImpl?wsdl)
Step8: If webservice is running from another system
(instead of localhost mention IP address)
Step9: After Locating WSDL, click on OK- Finish
Step 10: Now we our webservice client also ready.
Step 11: Now we need test this application, wether it is working or not, for that i
am creating simple Test class which will call the Proxy class and
internally it will call the webservice server and will give the response
to us.
Ex:
package com.siva;
import java.rmi.RemoteException;
public class Test {
public static void main(String[] args) {
ArthimeticServiceImplProxy proxy = new ArthimeticServiceImplProxy();
int result = 0;
try {
result = proxy.add(20, 30);
} catch (RemoteException e) {
e.printStackTrace();
}
System.out.println("result is.." + result);
}
}
}
Now you will get result as -- 50
Like this webservices will work.
If you want to call this webservice from JSP and servletes
Then write jsp - with two input paramaters
servlet- will access the two values and return the result after
calling the webservice.
Once everything is completed try this url from your system
http://localhost:8888/test_webservice_client/
then provide input values and click on the Get result then result will display on the screen.
Now you can elaborate what ever ypou like and do modifications for your requirement.
Now you can download the source code from the following link.
DOWNLOAD WEBSERVICE SERVER CODE
DOWNLOAD WEBSERVICE CLIENT CODE